Manage group permissions
Robust group chat permissions are key to providing users with a friendly and safe group chat experience.
Understand the group permissions system
Member statuses
Member statuses are the roles that can be assigned to each participant (inbox ID) in a group chat. These are the available member statuses:
- Member
- Everyone in a group chat is a member. A member can be granted admin or super admin status. If a member's admin or super admin status is removed, they are still a member of the group.
- Admin
- Super admin
Options
Use options to assign a role to a permission. These are the available options:
- All members
- Admin only
- Includes super admins
- Super admin only
Permissions
Permissions are the actions a group chat participant can be allowed to take. These are the available permissions:
- Grant admin status to a member
- Remove admin status from a member
- Add a member to the group
- Remove a member from the group
- Update group metadata, such as group name, description, and image
- Update group permissions on an item-by-item basis, such as calling
updateGroupNamePermission
orupdateAddMemberPermission
. To learn more, see Group.kt in the xmtp-android SDK repo.
The following permissions can be assigned by super admins only. This helps ensure that a “regular” admin cannot remove the super admin or otherwise destroy a group.
- Grant super admin status to a member
- Remove super admin status from a member
How the group permissions system works
When a group is created, all groups have the same permissions and options set:
- There is one super admin, and it is the group creator
- There are no admins
- Each user added to the group starts out as a member
The super admin has all of the available permissions and can use them to adjust the group’s permissions and options.
As the app developer, you can provide a UI that enables group participants to make further adjustments. For example, you can give the super admin the following options when creating the group:
- Add members
- Update group metadata
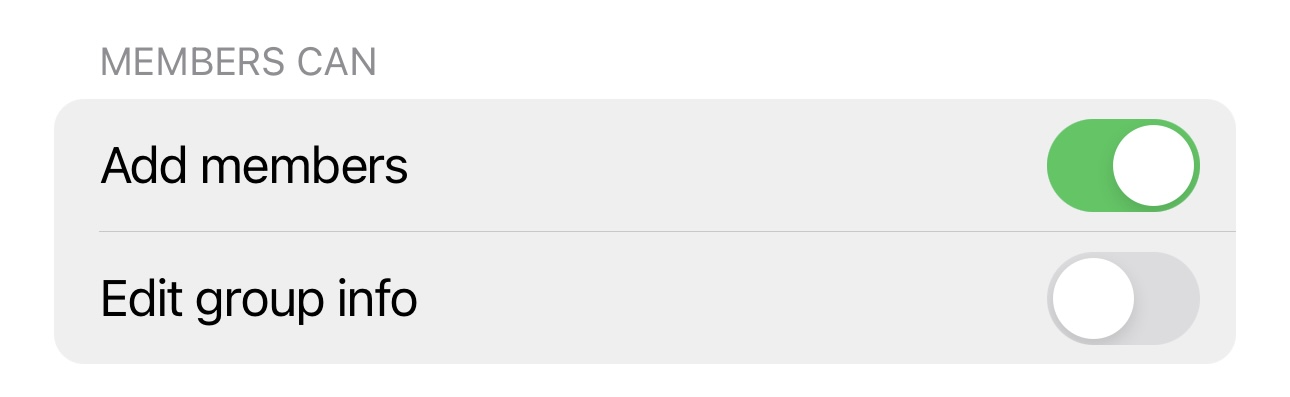
You can use member statuses, options, and permissions to create a custom policy set.
If you aren’t opinionated and don’t set any permissions and options, groups will default to using the delivered All_Members
policy set, which applies the following permissions and options:
- Add member - All members
- Remove member - Admin only
- Add admin - Super admin only
- Remove admin - Super admin only
- Update group permissions - Super admin only
- Update group metadata - All members
To learn more about the All_Members
and Admin_Only
policy sets, see group_permissions.rs in the LibXMTP repo.
Manage group chat admins
By design, checking admin permission status by wallet address is not supported. Instead, look up the inboxID
for that wallet address, then use the calls below.
Check if inbox ID is an admin
const isAdmin = group.isAdmin(inboxId);
Check if inbox ID is a super admin
const isSuperAdmin = group.isSuperAdmin(inboxId);
List admins
const admins = group.admins;
List super admins
const superAdmins = group.superAdmins;
Add admin status to inbox ID
await group.addAdmin(inboxId);
Add super admin status to inbox ID
await group.addSuperAdmin(inboxId);
Remove admin status from inbox ID
await group.removeAdmin(inboxId);
Remove super admin status from inbox ID
await group.removeSuperAdmin(inboxId);
Manage group chat membership
Add members by inbox ID
await group.addMembersByInboxId([inboxId]);
Add members by address
await group.addMembers([walletAddress]);
Remove members by inbox ID
await group.removeMembersByInboxId([inboxId]);
Remove members by address
await group.removeMembers([walletAddress]);
Get inbox IDs for members
const inboxId = await client.findInboxIdByAddress(address);
Get addresses for members
// sync group first
await group.sync();
// get group members
const members = await group.members();
// map inbox ID to account addresses
const inboxIdAddressMap = new Map(
members.map((member) => [member.inboxId, member.accountAddresses])
);
Get the inbox ID that added the current member
const addedByInboxId = group.addedByInboxId;
SDK method call flow to add and remove members
When you add or remove members from a group, a proposal/commit message is sent to all the installations in the group. When the commit happens, the cryptographic state of the group is changed. This allows new members to receive messages but not decrypt older ones and prevents removed members from decrypting messages after their departure.