Manage XMTP inboxes
The first time someone uses your app with a wallet address they've never used with any app built with XMTP, your app creates an inbox ID and installation ID associated with the wallet address. To do this, you create a client for their wallet address.
The client creates an inbox ID and installation ID associated with the wallet address. By default, this wallet address is designated as the recovery address. A recovery address will always have the same inbox ID and cannot be reassigned to a different inbox ID.
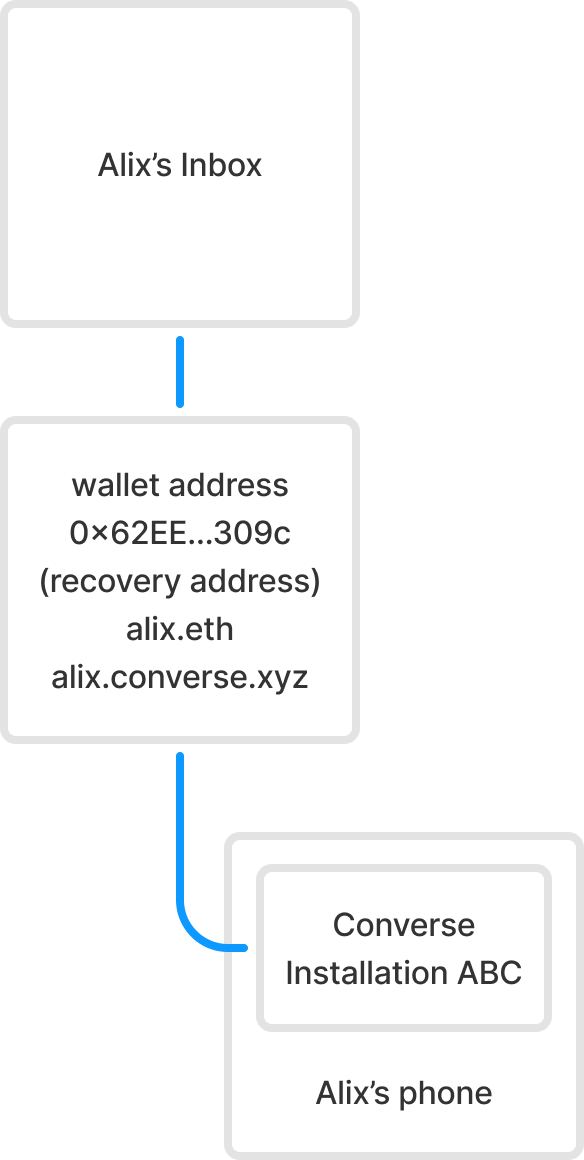
When you make subsequent calls to create a client for the same wallet address and a local database is not present, the client uses the same inbox ID, but creates a new installation ID.
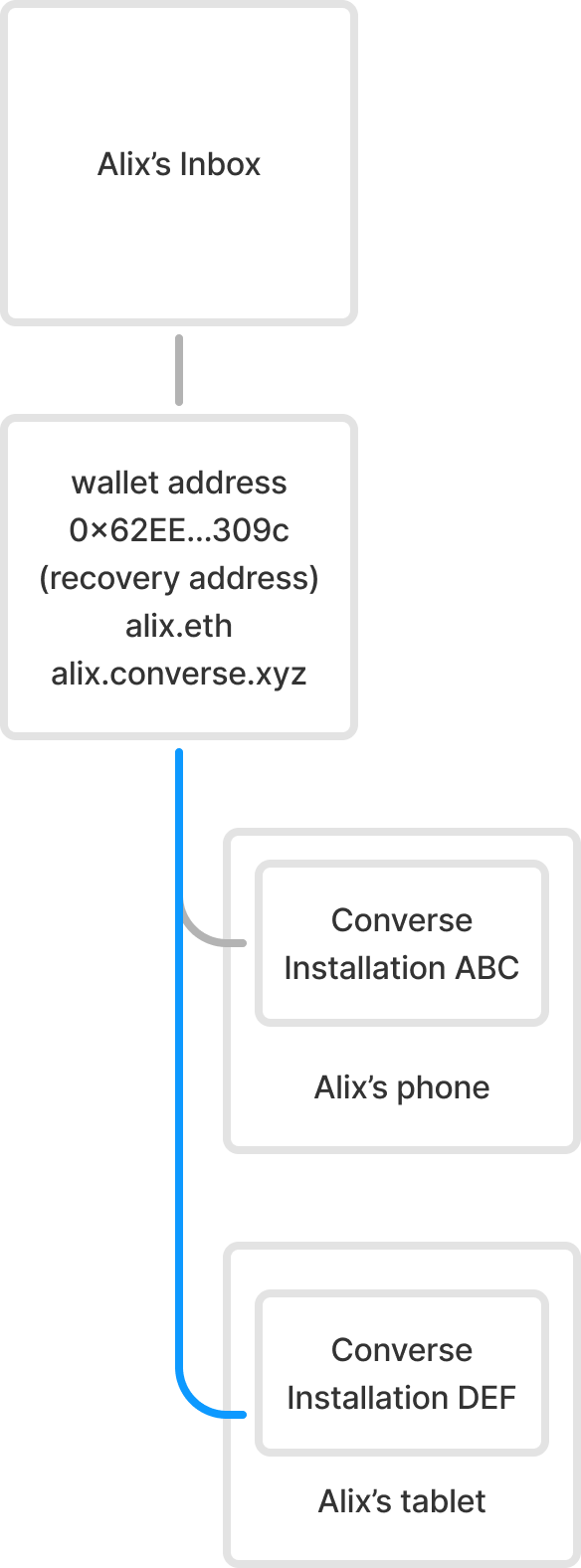
You can enable a user to add multiple wallet addresses to their inbox. Added addresses use the same inbox ID and the installation ID of the installation used to add the address.
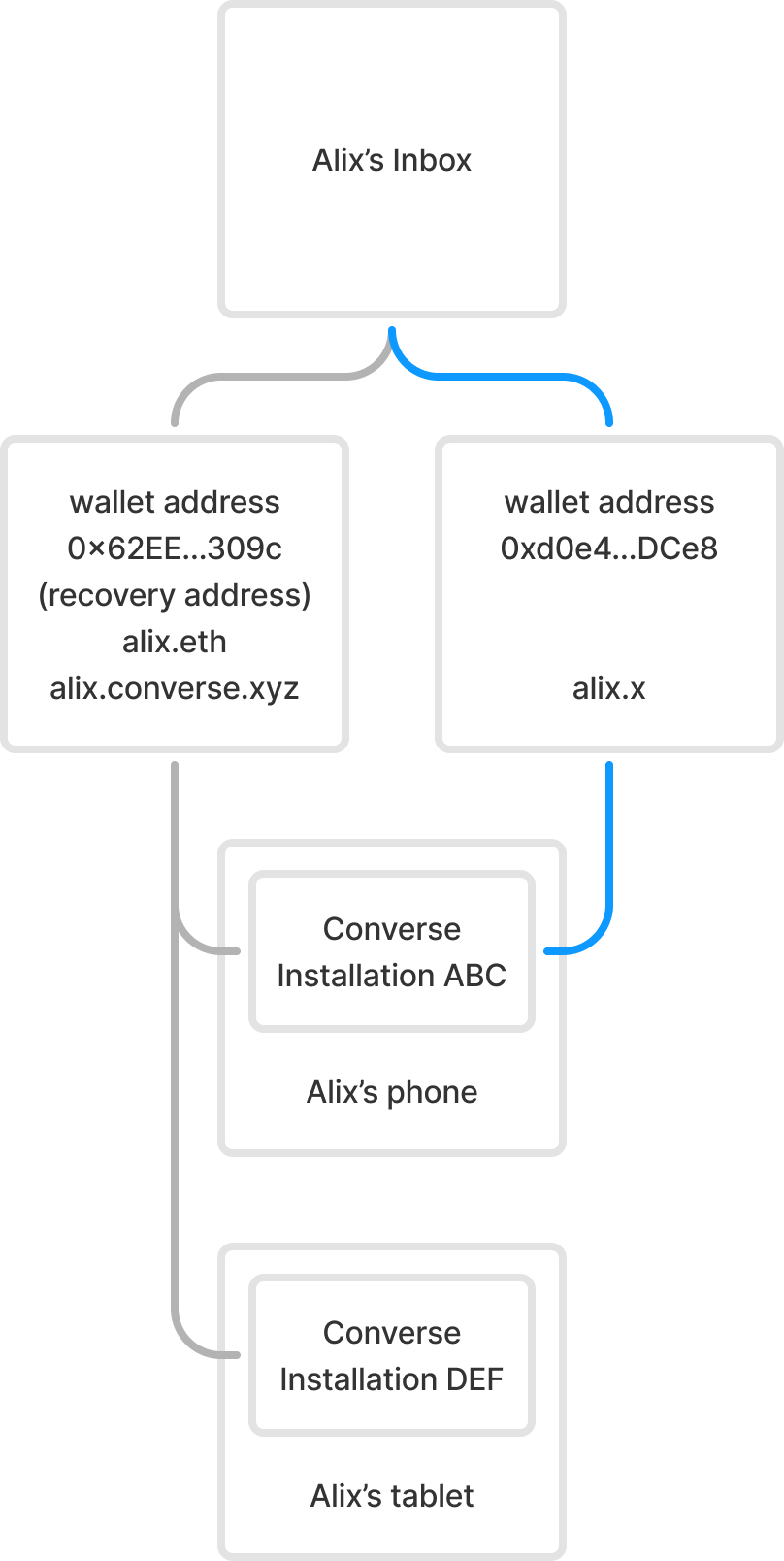
You can enable a user to remove a wallet address from their inbox. You cannot remove the recovery wallet address.
Add a wallet address to an inbox
await client.addAccount(walletToAdd)
Remove a wallet address from an inbox
await client.removeAccount(recoveryWallet, addressToRemove)
Revoke all other installations
You can revoke all installations other than the currently accessed installation.
For example, consider a user using this current installation:
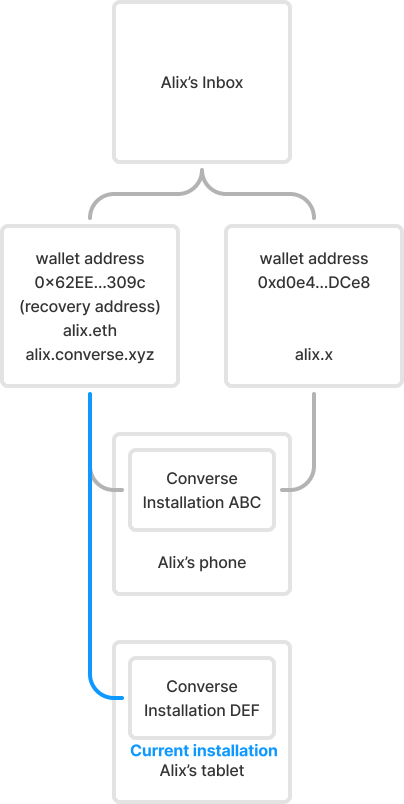
When the user revokes all other installations, the action removes all installations other than the current installation:
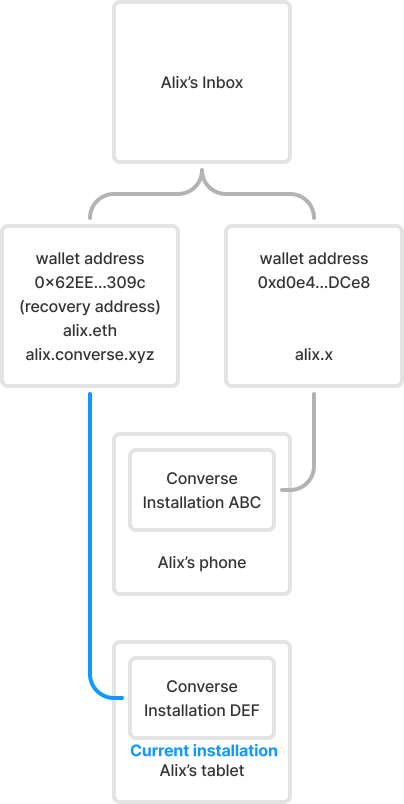
await client.revokeAllOtherInstallations(recoveryWallet)
View the inbox state
Find an inboxId
for an address:
const inboxId = await client.inboxIdFromAddress(address)
View the state of any inbox to see the addresses, installations, and other information associated with the inboxId
.
const state = await client.inboxState(true)
const states = await client.inboxStates(true, [inboxId, inboxId])
InboxState
{
recoveryAddress: string,
addresses: string[],
installations: string[],
inboxId: string,
}
FAQ
What happens when a user removes a wallet address?
Consider an inbox with four associated wallet addresses:
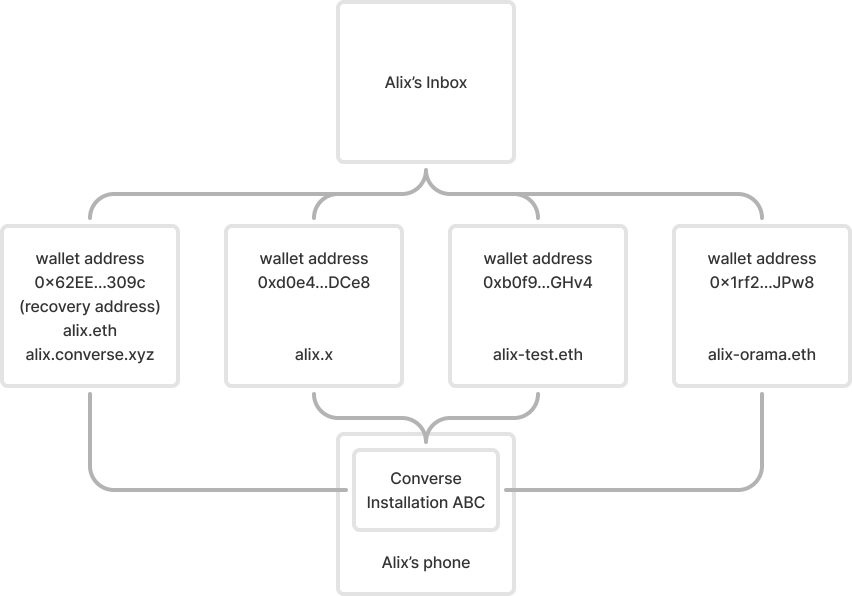
If the user removes an address from the inbox, the address no longer has access to the inbox it was removed from.
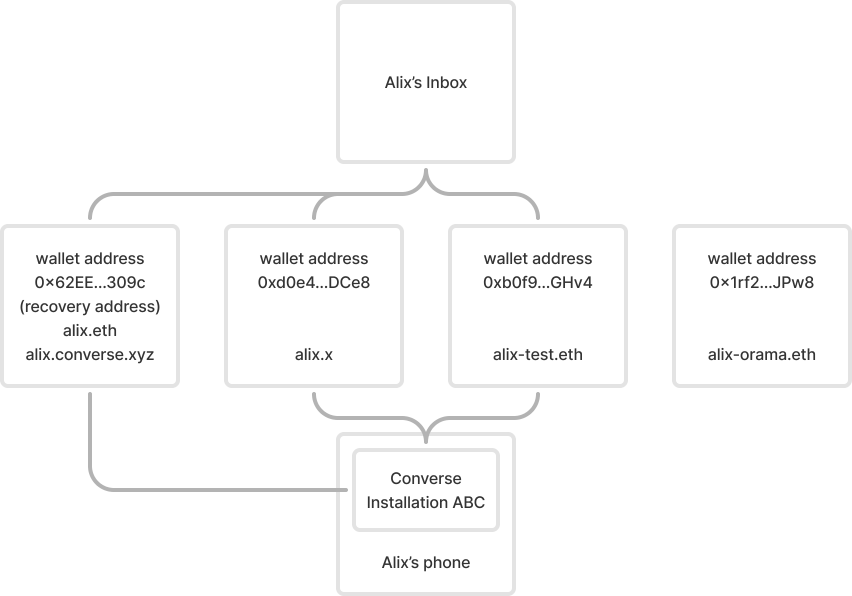
The address can no longer be added to or used to access conversations in that inbox. If someone sends a message to the address, the message is not associated with the original inbox. If the user logs in to a new installation with the address, this will create a new inbox ID.
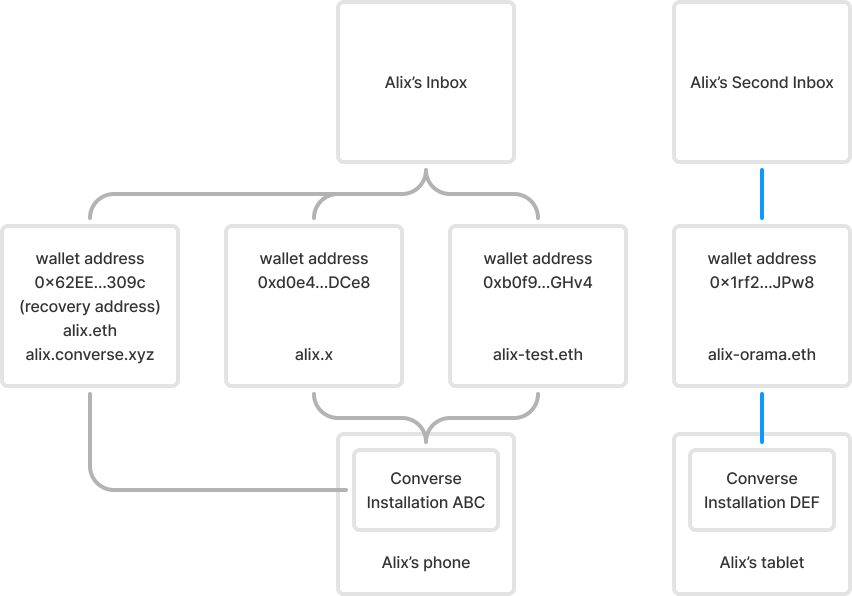
How is the recovery address used?
The recovery address and its signer can be used to sign transactions that remove addresses and revoke installations.
For example, Alix can give Bo access to their inbox so Bo can see their groups and conversations and respond for Alix.
If Alix decides they no longer want Bo have access to their inbox, Alix can use their recovery address signer to remove Bo.
However, while Bo has access to Alix's inbox, Bo cannot remove Alix from their own inbox because Bo does not have access to Alix's recovery address signer.
If a user created two inboxes using two wallet addresses, is there a way to combine the inboxes?
If a user logs in with wallet address 0x62EE...309c and creates inbox 1 and then logs in with wallet address 0xd0e4...DCe8 and creates inbox 2; there is no way to combine inbox 1 and 2.
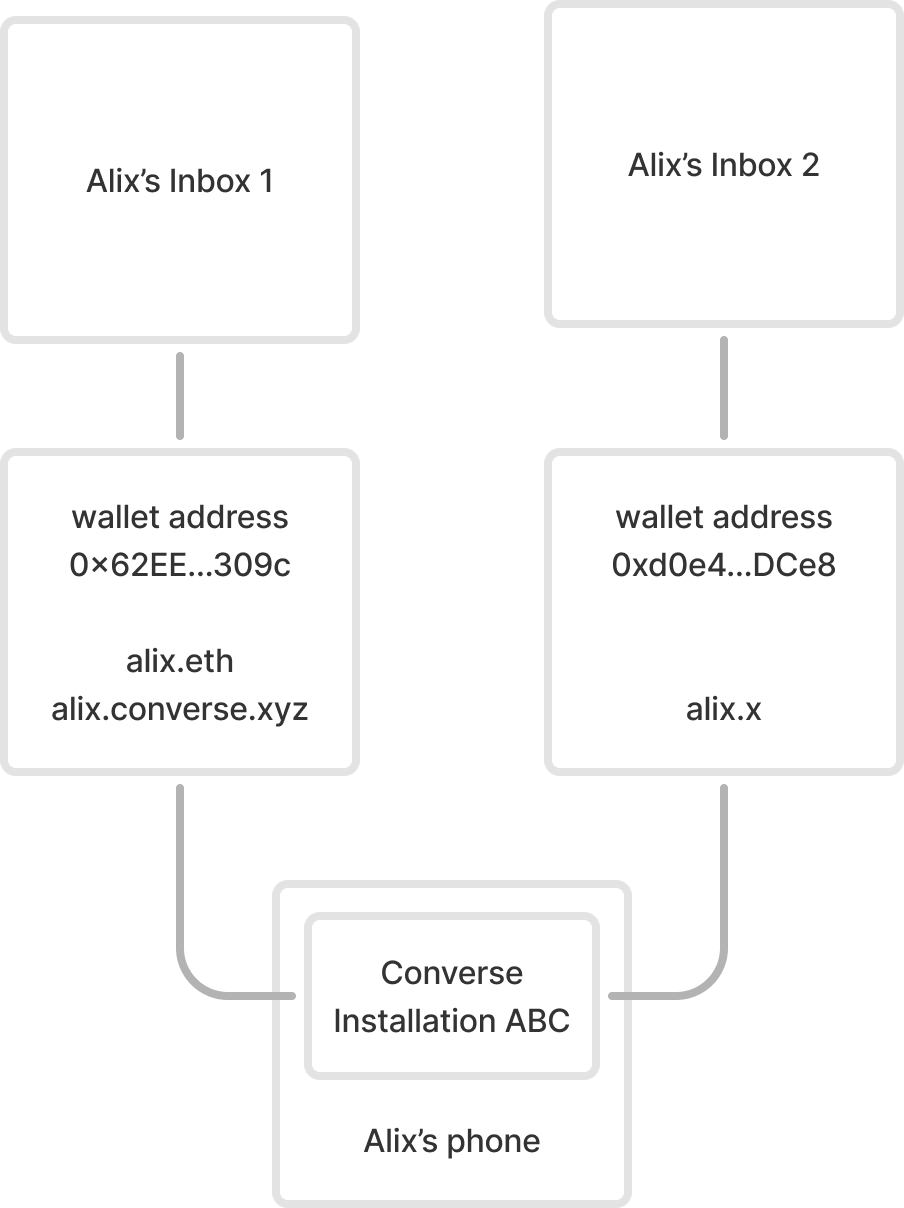
You can add wallet address 0xd0e4...DCe8 to inbox 1, but both address 0x62EE...309c and 0xd0e4...DCe8 would then have access to inbox 1 only. Wallet address 0xd0e4...DCe8 would no longer be able to access inbox 2.
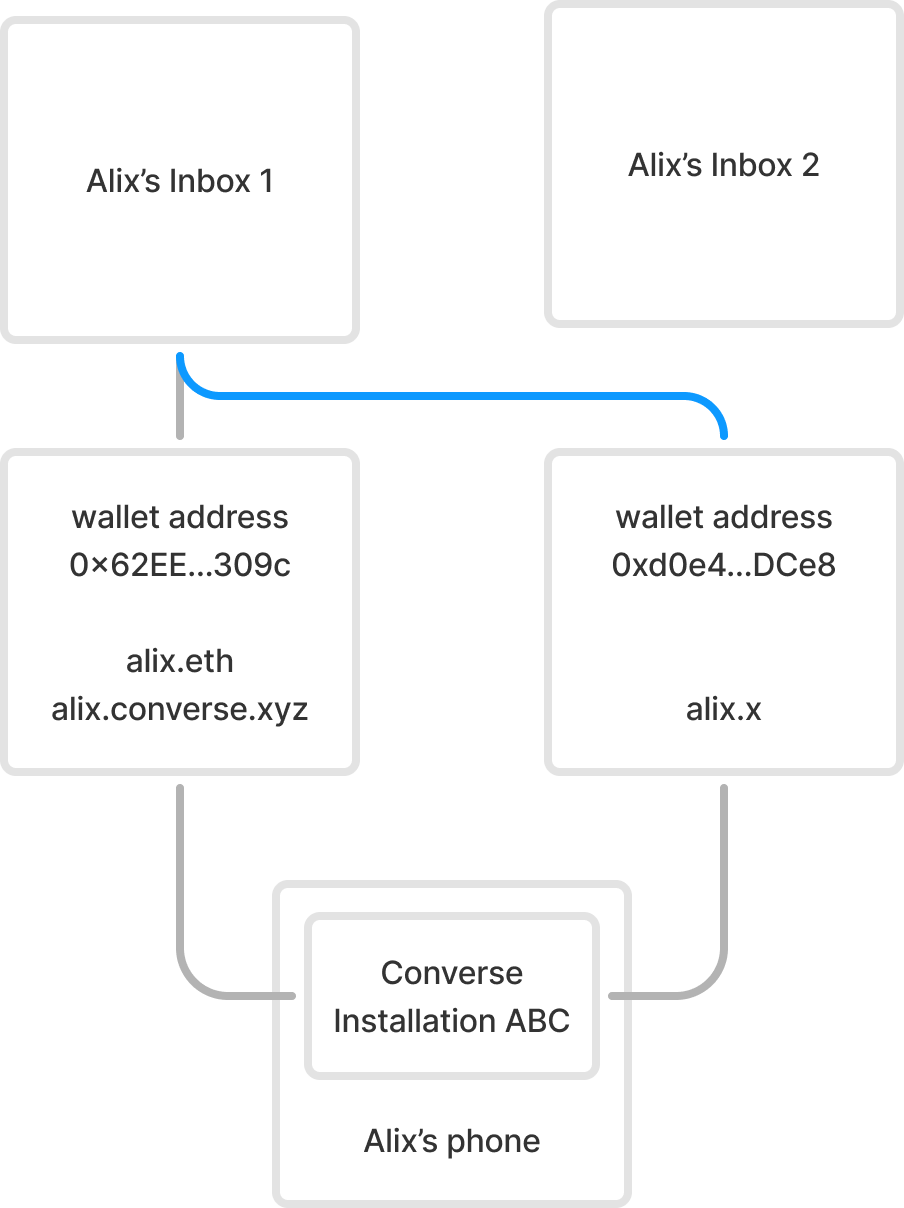
To help users avoid this state, ensure that your UX surfaces their ability to add multiple wallet addresses to a single inbox.
What happens if I remove a wallet address from an inbox ID and then initiate a client with the private key of the removed address?
Does the client create a new inbox ID or does it match it with the original inbox ID the address was removed from?The wallet address used to initiate a client should be matched to its original inbox ID.
You do have the ability to rotate inbox IDs if a user reaches the limit of 257 identity actions (adding, removing, or revoking addresses or installations). Hopefully, users won’t reach this limit, but if they do, inbox IDs have a nonce and can be created an infinite number of times.
However, anytime a new inbox ID is created for an address, the conversations and messages in any existing inbox ID associated with the address are lost.